Slice: Extracts a section of an array and returns a new array. Splice: Changes the content of an array by removing, replacing, or adding elements. In JavaScript, these are two commonly used methods when it comes to manipulating arrays. These methods may sound similar, but they have distinct functionalities and use cases. It’s crucial to understand the differences between them to utilize them effectively in your code. In this article “Slice vs Splice in JavaScript”, we’ll explore the methods, examine their characteristics, and provide practical examples to illustrate their usage.
Let’s explore further
Contents
The slice() Method
The slice() method is mainly used to create a shallow copy of a portion of an array. It extracts elements from an array and returns them as a new array without modifying the original array. This method takes two parameters: the starting index and the optional ending index. It includes the element at the starting index and excludes the element at the ending index, creating a new array with the selected elements.
Here’s the syntax for the slice() method:
array.slice(startIndex, endIndex);
- startIndex (required): The index at which to begin extraction. If negative, it counts from the end of the array.
- endIndex (optional): The index at which to stop extraction. This element is not included in the returned array. If omitted, slice() extracts until the end of the array. If negative, it counts from the end of the array.
Slice: Examples
Let’s explore some examples to better understand the slice() method:
const fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry'];
const slicedFruits = fruits.slice(1, 4);
console.log(slicedFruits);
// Output: ['banana', 'cherry', 'date']
const slicedFruits2 = fruits.slice(2);
console.log(slicedFruits2);
// Output: ['cherry', 'date', 'elderberry']
const slicedFruits3 = fruits.slice(-2);
console.log(slicedFruits3);
// Output: ['date', 'elderberry']
const slicedFruits4 = fruits.slice(1, -1);
console.log(slicedFruits4);
// Output: ['banana', 'cherry', 'date']
In the first example, fruits.slice(1, 4) extracts elements from index 1 to index 3 (‘banana’, ‘cherry’, ‘date’), but does not include the element at index 4 (‘elderberry’).
The second example, fruits.slice(2), starts extraction from index 2 and includes all the remaining elements ([‘cherry’, ‘date’, ‘elderberry’]).
The third example demonstrates the use of negative indices. fruits.slice(-2) extracts the last two elements of the array ([‘date’, ‘elderberry’]).
Lastly, fruits.slice(1, -1) excludes the first and last elements ([‘banana’, ‘cherry’, ‘date’]).
Slice: Visual Example
Let’s take the first example of fruits array and visualize how the slice operation changes the array.
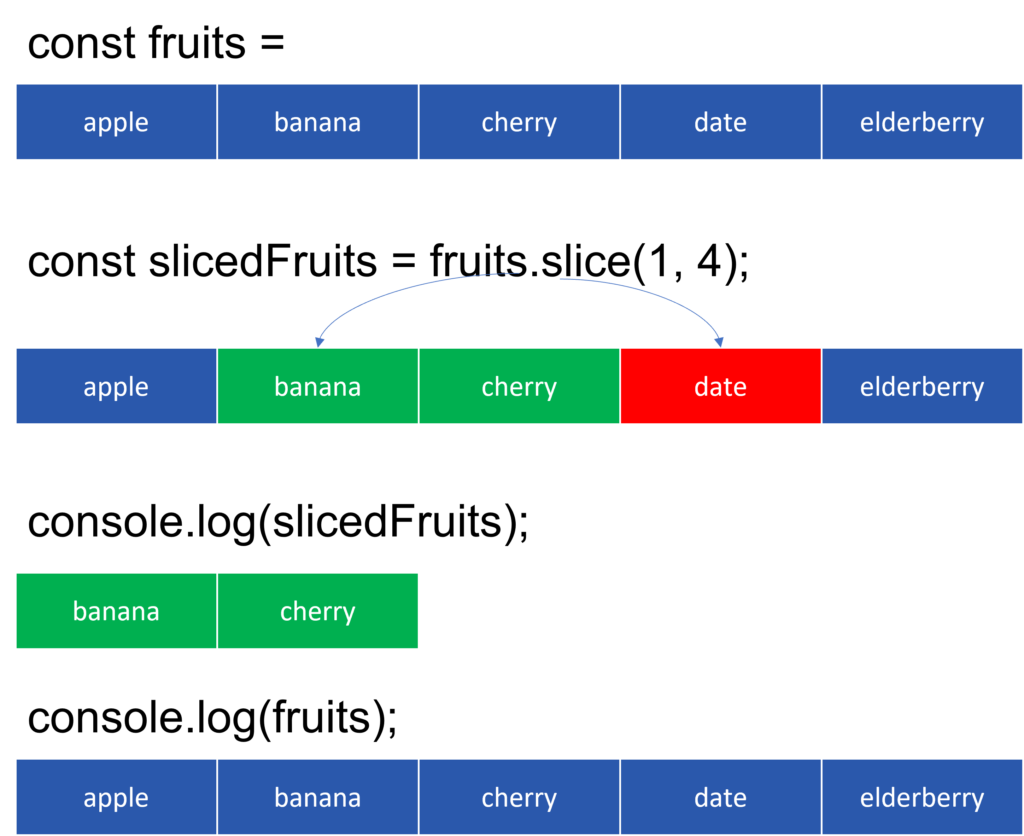
Now lets move on to the next method:
The splice() Method
While the slice() method extracts a portion of an array, the splice() method is used for both extracting and adding elements to an array. It modifies the original array and returns the removed elements as a new array. With splice(), you can remove, replace, or insert elements at specific positions within an array.
Here’s the syntax for the splice() method:
array.splice(startIndex, deleteCount, item1, item2, ...);
- startIndex (required): The index at which to start modifying the array. If negative, it counts from the end of the array.
- deleteCount (optional): The number of elements to remove from the array. If set to 0, no elements are removed.
- item1, item2, … (optional): Elements to be added to the array at the startIndex.
Let’s explore some examples to better understand the splice() method:
const numbers = [1, 2, 3, 4, 5];
const removedElements = numbers.splice(2, 2);
console.log(removedElements);
// Output: [3, 4]
console.log(numbers);
// Output: [1, 2, 5]
numbers.splice(1, 0, 'a', 'b');
console.log(numbers);
// Output: [1, 'a', 'b', 2, 5]
numbers.splice(3, 1, 'c', 'd');
console.log(numbers);
// Output: [1, 'a', 'b', 'c', 'd', 5]
In the first example, numbers.splice(2, 2) removes two elements starting from index 2 (3 and 4) and returns them as an array. After the operation, numbers is modified to [1, 2, 5], as the elements at index 2 and 3 are removed.
The second example, numbers.splice(1, 0, ‘a’, ‘b’), inserts the elements ‘a’ and ‘b’ at index 1 without removing any elements. The resulting array is [1, ‘a’, ‘b’, 2, 5].
In the third example, numbers.splice(3, 1, ‘c’, ‘d’) replaces one element at index 3 with ‘c’ and ‘d’. The resulting array is [1, ‘a’, ‘b’, ‘c’, ‘d’, 5].
Splice: Visual Example
Let’s take the second example of numbers array and visualize how the splice operation changes the array. From the First Example we have the new updated array after removing elements as [1,2,5]. Now we apply numbers.splice(1, 0, ‘a’, ‘b’);
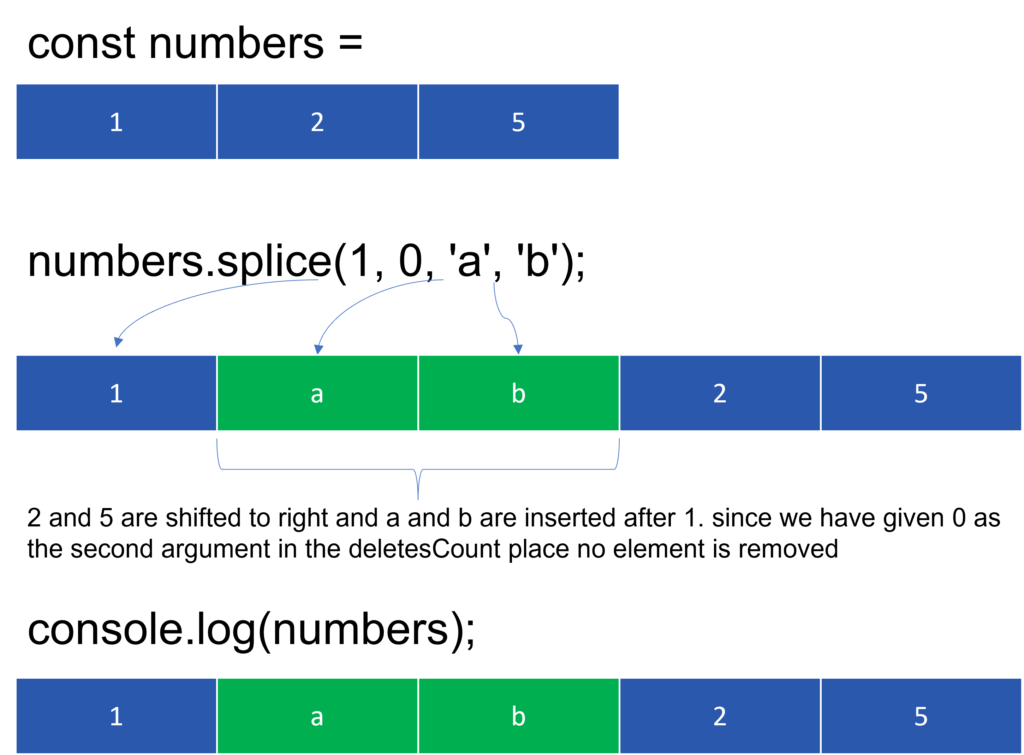
Summary
Understanding the differences between the slice() and splice() methods in JavaScript is essential for effective array manipulation. While slice() creates a new array by extracting a portion of an existing array, splice() modifies the original array by removing or adding elements. By mastering these methods, you can confidently manipulate arrays in your JavaScript code, making your programs more dynamic and flexible. If you have found the article useful kindly share it with your friends and follow us on our social media platforms (Instagram, twitter)
Read about other JavaScript Array Methods: https://deepakjosecodes.com/category/javascript/
Comment down your thoughts and suggestions for future posts
Our other Articles
- Ultimate Data Science Roadmap: One Guide To Rule Them All
- Data Science Career Paths & Tips To Choose The Right One
- Difference Between a Hash Table and a Dictionary
- Most Frequently Asked Coding Interview Questions And Answers
- How To Get Coursera Courses With Certificate For Free
- Unique Machine Learning Project Ideas To Build An Impressive Portfolio
- Essential Python/Machine Learning Libraries And Resources To Master Them
- How To Debug Code Efficiently: Tips And Tricks
- What Is CORS? Why Is It Important? How To Use IT In A MEAN App?