Push, Pop, Shift, and Unshift are JavaScript Array Methods used for Array Manipulation.
- push: Adds one or more elements to the end of an array.
- pop: Removes the last element from an array and returns it.
- shift: Removes the first element from an array and returns it.
- unshift: Adds one or more elements to the beginning of an array.
Let’s see these methods in action:
Contents
Push() in JavaScript Array Methods
push() method in JavaScript is used to add an element to the end of an array.
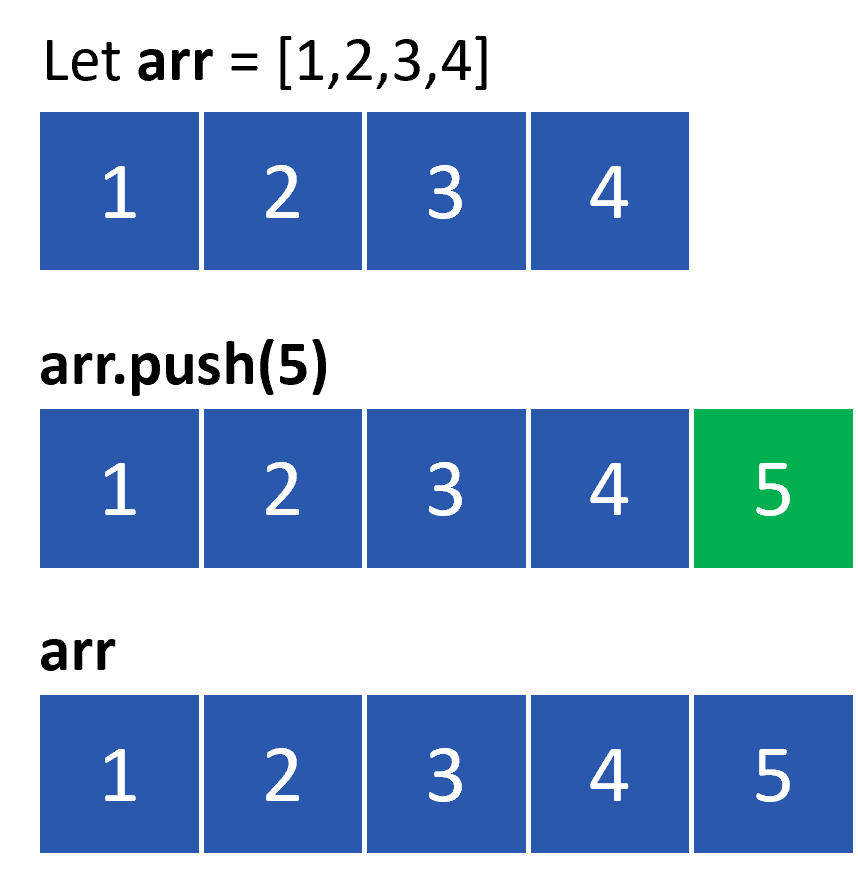
Code Example
// Create an array
let arr = [1, 2, 3, 4];
// Add `5` to the end using `push`
arr.push(5);
console.log(arr); // Output: [1, 2, 3, 4, 5]
Pop() in JavaScript Array Methods
The pop() method in JavaScript is used to remove an element from the end of an array.
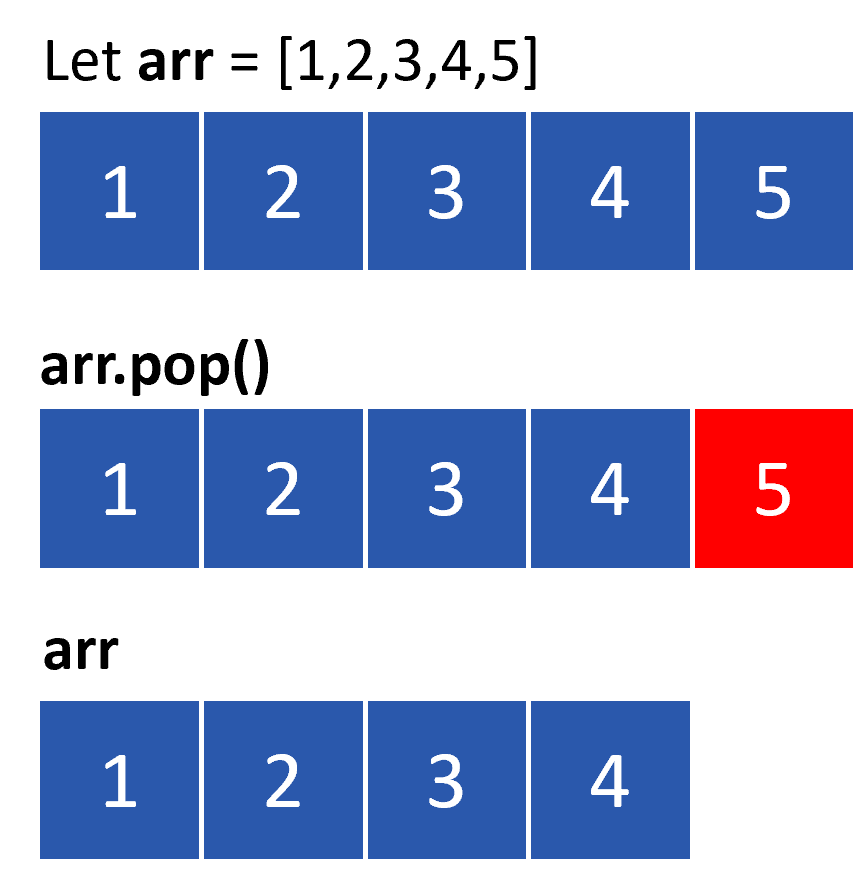
Code Example
// Create an array
let arr = [1, 2, 3, 4, 5];
const lastRemoved = arr.pop();
console.log(arr); // Output: [1, 2, 3, 4]
console.log(lastRemoved); // Output: 5
Shift() in JavaScript Array methods
shift() method in JavaScript is used to remove an element from the beginning of an array.
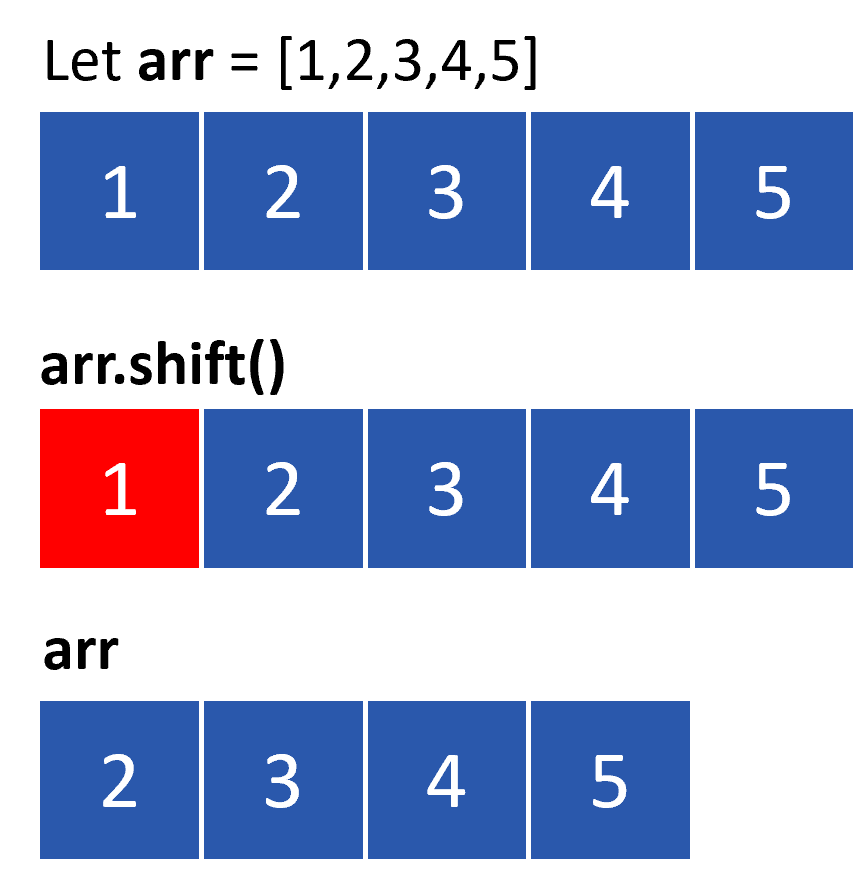
Code Example
let arr = [1, 2, 3, 4, 5];
const firstRemoved = arr.shift();
console.log(arr); // Output: [2, 3, 4, 5]
console.log(firstRemoved); // Output: 1
Unshift() in JavaScript Array methods
unshift() method in JavaScript is used to remove an element from the beginning of an array.
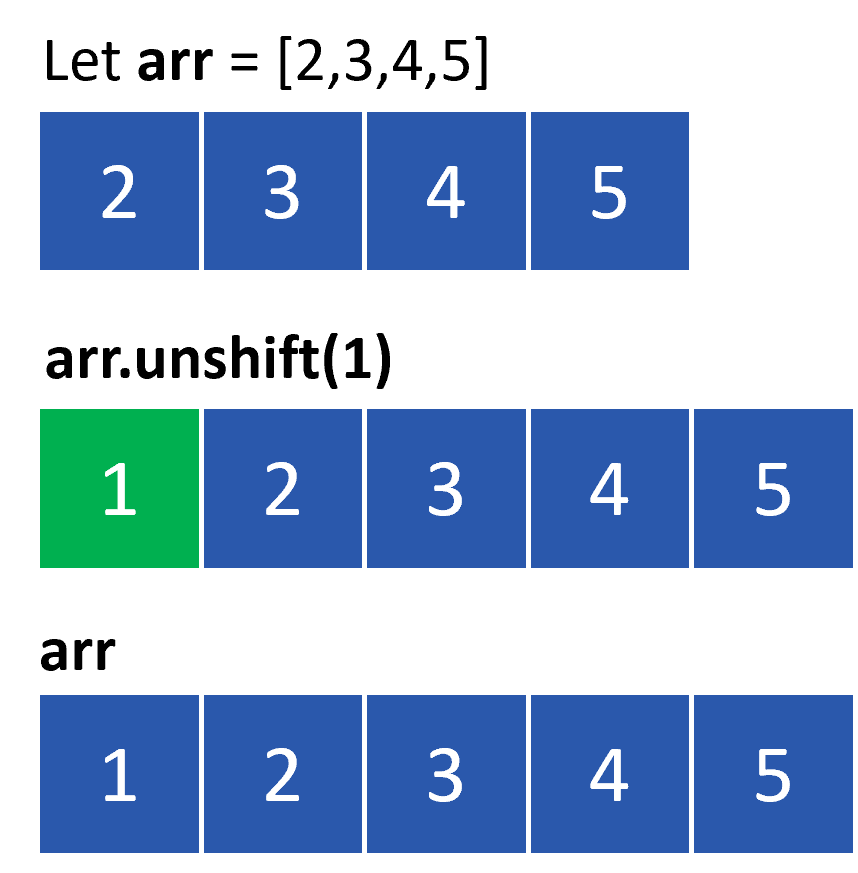
Code Example
let arr = [2, 3, 4, 5];
// Add `1` at the beginning using `unshift`
arr.unshift(1);
console.log(arr); // Output: [1, 2, 3, 4, 5]
Bonus:
- Adding multiple elements:
push
andunshift
accept multiple arguments, allowing you to add several elements at once. - Performance:
push
andpop
are generally faster thanshift
andunshift
, as they avoid shifting elements within the array. - Chaining: You can chain these methods together for complex manipulations, but exercise caution to avoid unexpected results.
When to Use Each Method:
- Use push to add elements to the end for building stacks or queues.
- Use pop to remove and access the last element, ideal for undo/redo functionality.
- shift to remove and access the first element, useful for processing FIFO (First-In-First-Out) data.
- unshift to add elements to the beginning, like creating a playlist or reversing an array.
Remember: These methods modify the original array, so be mindful if you need to preserve a copy. For more complex modifications, consider using methods like splice and slice.
Conclusion
I hope this article provides a clear and informative guide to JavaScript array manipulation using push, pop, shift, and unshift. Mastering these methods empowers you to work with dynamic data in JavaScript with confidence and efficiency. So, practice, experiment, and unlock the full potential of your arrays! Read JavaScript Official Documentation to learn more
Also Read: https://deepakjosecodes.com/slice-vs-splice-in-javascript-a-comprehensive-comparison/
Comment down your thoughts and suggestions for future posts
